Let’s say you have two main categories for your posts:
- Europe
- Asia
And posts from these main categories also have one or more of the same additional categories.
- Europe
- Flights
- Hotels
- Dining
- Asia
- Flights
- Hotels
- Dining
Now lets say you only want to include posts that have “Europe” as a category, but also only want to include “Europe” posts with either “Flights” or “Hotels”, and NOT “Dining”.
So the logic would be:
(Europe AND Flights) OR (Europe AND Hotels)
This can be accomplished by hooking into one of the plugin’s PHP filters.
Step 1:
For the above scenario, choose “Europe”, “Flights” and “Hotels” as your Categories, and then choose “OR” for the Category Relation.
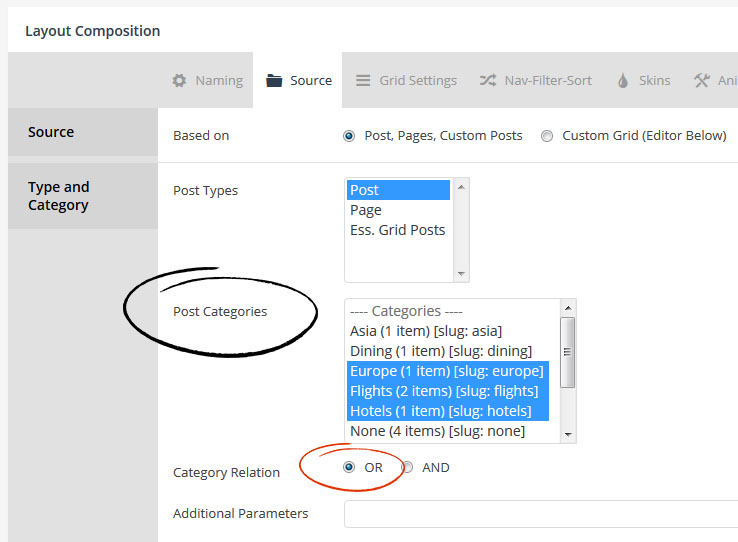
Step 2:
Next, add the following PHP code to your theme’s “functions.php” file.
In the following code, the number “121” is the Category ID for “Europe”. Adjust this number accordingly.
add_filter('essgrid_modify_posts', 'eg_modify_post', 10, 2);
function eg_modify_post($posts, $grid_id){
$filterPosts = array();
foreach($posts as $post) {
foreach($post['post_category'] as $cat) {
// where "121" is the Category ID or "Europe"
if($cat === 121) {
$filterPosts[] = $post;
break;
}
}
}
return $filterPosts;
}
Variation of above code for WooCommerce Product Categories
add_filter('essgrid_modify_posts', 'eg_modify_post', 10, 2);
function eg_modify_post($posts, $grid_id) {
$filterPosts = array();
foreach($posts as $post) {
$cats = get_the_terms($post['ID'], 'product_cat');
foreach($cats as $cat) {
// where "121" is the Category ID or "Europe"
if($cat->term_id === 121) {
$filterPosts[] = $post;
break;
}
}
}
return $filterPosts;
}
Variation to include Sub Categories
add_filter('essgrid_modify_posts', 'eg_modify_post', 10, 2);
function eg_modify_post($posts, $grid_id){
$filterPosts = array();
foreach($posts as $post) {
$cats = $post['post_category'];
foreach($cats as $cat) {
// parent-category ID is "25" or "26"
if($cat === 25 || $cat === 26) {
$subs = get_categories(array('child_of' => $cat));
foreach($subs as $sub) {
$id = $sub -> cat_ID;
if(in_array($id, $cats)) {
// sub-category ID is "27" or "28"
if($id === 27 || $id === 28) {
$filterPosts[] = $post;
}
}
}
}
}
}
return $filterPosts;
}